[lld] [LLD][COFF] Add support for `--time-trace` (PR #68236)
via llvm-commits
llvm-commits at lists.llvm.org
Wed Oct 4 10:28:53 PDT 2023
llvmbot wrote:
<!--LLVM PR SUMMARY COMMENT-->
@llvm/pr-subscribers-lld-coff
<details>
<summary>Changes</summary>
This adds support for generating Chrome-tracing .json profile traces.
Also add the necessary time scopes, so that the profile trace shows in great detail which tasks are executed.
As an example, this is what we see when linking a Unreal Engine executable:
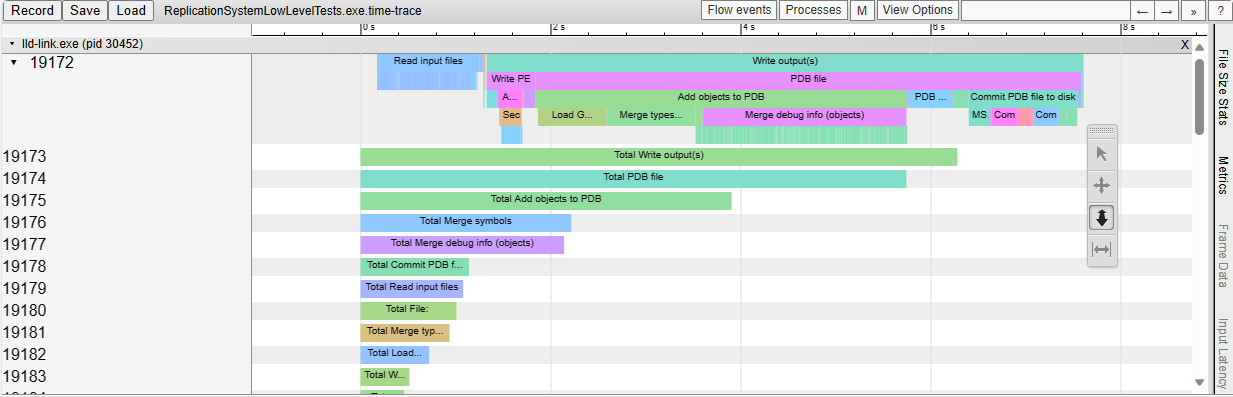
---
Patch is 49.83 KiB, truncated to 20.00 KiB below, full version: https://github.com/llvm/llvm-project/pull/68236.diff
25 Files Affected:
- (modified) lld/COFF/Config.h (+2)
- (modified) lld/COFF/DebugTypes.cpp (+3)
- (modified) lld/COFF/Driver.cpp (+120-78)
- (modified) lld/COFF/DriverUtils.cpp (+2)
- (modified) lld/COFF/ICF.cpp (+2)
- (modified) lld/COFF/LLDMapFile.cpp (+2)
- (modified) lld/COFF/LTO.cpp (+2)
- (modified) lld/COFF/MapFile.cpp (+2)
- (modified) lld/COFF/MarkLive.cpp (+2)
- (modified) lld/COFF/MinGW.cpp (+2)
- (modified) lld/COFF/Options.td (+8)
- (modified) lld/COFF/PDB.cpp (+58-33)
- (modified) lld/COFF/SymbolTable.cpp (+2)
- (modified) lld/COFF/Writer.cpp (+82-43)
- (modified) lld/Common/Filesystem.cpp (+2)
- (modified) lld/docs/ReleaseNotes.rst (+3)
- (added) lld/test/COFF/time-trace.s (+44)
- (modified) llvm/lib/DebugInfo/MSF/MSFBuilder.cpp (+5)
- (modified) llvm/lib/DebugInfo/PDB/Native/DbiStreamBuilder.cpp (+2)
- (modified) llvm/lib/DebugInfo/PDB/Native/GSIStreamBuilder.cpp (+2)
- (modified) llvm/lib/DebugInfo/PDB/Native/InfoStreamBuilder.cpp (+2)
- (modified) llvm/lib/DebugInfo/PDB/Native/PDBFileBuilder.cpp (+17-9)
- (modified) llvm/lib/DebugInfo/PDB/Native/PDBStringTableBuilder.cpp (+2)
- (modified) llvm/lib/DebugInfo/PDB/Native/TpiStreamBuilder.cpp (+2)
- (modified) llvm/lib/Support/FileOutputBuffer.cpp (+3)
``````````diff
diff --git a/lld/COFF/Config.h b/lld/COFF/Config.h
index da31818be23dc1e..2d5d4d63d69fa4a 100644
--- a/lld/COFF/Config.h
+++ b/lld/COFF/Config.h
@@ -286,6 +286,7 @@ struct Configuration {
uint32_t minorSubsystemVersion = 0;
uint32_t timestamp = 0;
uint32_t functionPadMin = 0;
+ uint32_t timeTraceGranularity = 0;
bool dynamicBase = true;
bool allowBind = true;
bool cetCompat = false;
@@ -309,6 +310,7 @@ struct Configuration {
bool swaprunNet = false;
bool thinLTOEmitImportsFiles;
bool thinLTOIndexOnly;
+ bool timeTraceEnabled = false;
bool autoImport = false;
bool pseudoRelocs = false;
bool stdcallFixup = false;
diff --git a/lld/COFF/DebugTypes.cpp b/lld/COFF/DebugTypes.cpp
index 23ddccea695f884..5071b7b79d23e3f 100644
--- a/lld/COFF/DebugTypes.cpp
+++ b/lld/COFF/DebugTypes.cpp
@@ -29,6 +29,7 @@
#include "llvm/Support/FormatVariadic.h"
#include "llvm/Support/Parallel.h"
#include "llvm/Support/Path.h"
+#include "llvm/Support/TimeProfiler.h"
using namespace llvm;
using namespace llvm::codeview;
@@ -1068,6 +1069,7 @@ TypeMerger::~TypeMerger() = default;
void TypeMerger::mergeTypesWithGHash() {
// Load ghashes. Do type servers and PCH objects first.
{
+ llvm::TimeTraceScope timeScope("Load GHASHes");
ScopedTimer t1(ctx.loadGHashTimer);
parallelForEach(dependencySources,
[&](TpiSource *source) { source->loadGHashes(); });
@@ -1075,6 +1077,7 @@ void TypeMerger::mergeTypesWithGHash() {
[&](TpiSource *source) { source->loadGHashes(); });
}
+ llvm::TimeTraceScope timeScope("Merge types (GHASH)");
ScopedTimer t2(ctx.mergeGHashTimer);
GHashState ghashState;
diff --git a/lld/COFF/Driver.cpp b/lld/COFF/Driver.cpp
index 554db5969ea13dd..c6a7be14017fe7f 100644
--- a/lld/COFF/Driver.cpp
+++ b/lld/COFF/Driver.cpp
@@ -247,6 +247,7 @@ void LinkerDriver::enqueuePath(StringRef path, bool wholeArchive, bool lazy) {
createFutureForFile(std::string(path)));
std::string pathStr = std::string(path);
enqueueTask([=]() {
+ llvm::TimeTraceScope timeScope("File: ", path);
auto [mb, ec] = future->get();
if (ec) {
// Retry reading the file (synchronously) now that we may have added
@@ -332,6 +333,7 @@ void LinkerDriver::enqueueArchiveMember(const Archive::Child &c,
reportBufferError(mbOrErr.takeError(), check(c.getFullName()));
MemoryBufferRef mb = mbOrErr.get();
enqueueTask([=]() {
+ llvm::TimeTraceScope timeScope("Archive: ", mb.getBufferIdentifier());
ctx.driver.addArchiveBuffer(mb, toCOFFString(ctx, sym), parentName,
offsetInArchive);
});
@@ -348,6 +350,8 @@ void LinkerDriver::enqueueArchiveMember(const Archive::Child &c,
auto mbOrErr = future->get();
if (mbOrErr.second)
reportBufferError(errorCodeToError(mbOrErr.second), childName);
+ llvm::TimeTraceScope timeScope("Archive: ",
+ mbOrErr.first->getBufferIdentifier());
// Pass empty string as archive name so that the original filename is
// used as the buffer identifier.
ctx.driver.addArchiveBuffer(takeBuffer(std::move(mbOrErr.first)),
@@ -974,6 +978,7 @@ std::string LinkerDriver::getImportName(bool asLib) {
}
void LinkerDriver::createImportLibrary(bool asLib) {
+ llvm::TimeTraceScope timeScope("Create import library");
std::vector<COFFShortExport> exports;
for (Export &e1 : ctx.config.exports) {
COFFShortExport e2;
@@ -1031,6 +1036,7 @@ void LinkerDriver::createImportLibrary(bool asLib) {
}
void LinkerDriver::parseModuleDefs(StringRef path) {
+ llvm::TimeTraceScope timeScope("Parse def file");
std::unique_ptr<MemoryBuffer> mb =
CHECK(MemoryBuffer::getFile(path, /*IsText=*/false,
/*RequiresNullTerminator=*/false,
@@ -1095,6 +1101,7 @@ void LinkerDriver::enqueueTask(std::function<void()> task) {
}
bool LinkerDriver::run() {
+ llvm::TimeTraceScope timeScope("Read input files");
ScopedTimer t(ctx.inputFileTimer);
bool didWork = !taskQueue.empty();
@@ -1233,6 +1240,8 @@ static void markAddrsig(Symbol *s) {
}
static void findKeepUniqueSections(COFFLinkerContext &ctx) {
+ llvm::TimeTraceScope timeScope("Find keep unique sections");
+
// Exported symbols could be address-significant in other executables or DSOs,
// so we conservatively mark them as address-significant.
for (Export &r : ctx.config.exports)
@@ -1325,6 +1334,7 @@ void LinkerDriver::parsePDBAltPath() {
/// trees into one resource tree.
/// Call after ObjFile::Instances is complete.
void LinkerDriver::convertResources() {
+ llvm::TimeTraceScope timeScope("Convert resources");
std::vector<ObjFile *> resourceObjFiles;
for (ObjFile *f : ctx.objFileInstances) {
@@ -1478,6 +1488,16 @@ void LinkerDriver::linkerMain(ArrayRef<const char *> argsArr) {
ArgParser parser(ctx);
opt::InputArgList args = parser.parse(argsArr);
+ // Initialize time trace profiler.
+ config->timeTraceEnabled = args.hasArg(OPT_time_trace_eq);
+ config->timeTraceGranularity =
+ args::getInteger(args, OPT_time_trace_granularity_eq, 500);
+
+ if (config->timeTraceEnabled)
+ timeTraceProfilerInitialize(config->timeTraceGranularity, argsArr[0]);
+
+ llvm::TimeTraceScope timeScope("COFF link");
+
// Parse and evaluate -mllvm options.
std::vector<const char *> v;
v.push_back("lld-link (LLVM option parsing)");
@@ -2127,6 +2147,7 @@ void LinkerDriver::linkerMain(ArrayRef<const char *> argsArr) {
parseFunctionPadMin(arg);
if (tar) {
+ llvm::TimeTraceScope timeScope("Reproducer: response file");
tar->append("response.txt",
createResponseFile(args, filePaths,
ArrayRef<StringRef>(searchPaths).slice(1)));
@@ -2147,15 +2168,18 @@ void LinkerDriver::linkerMain(ArrayRef<const char *> argsArr) {
machineToStr(config->machine));
// Handle /export
- for (auto *arg : args.filtered(OPT_export)) {
- Export e = parseExport(arg->getValue());
- if (config->machine == I386) {
- if (!isDecorated(e.name))
- e.name = saver().save("_" + e.name);
- if (!e.extName.empty() && !isDecorated(e.extName))
- e.extName = saver().save("_" + e.extName);
+ {
+ llvm::TimeTraceScope timeScope("Parse /export");
+ for (auto *arg : args.filtered(OPT_export)) {
+ Export e = parseExport(arg->getValue());
+ if (config->machine == I386) {
+ if (!isDecorated(e.name))
+ e.name = saver().save("_" + e.name);
+ if (!e.extName.empty() && !isDecorated(e.extName))
+ e.extName = saver().save("_" + e.extName);
+ }
+ config->exports.push_back(e);
}
- config->exports.push_back(e);
}
// Handle /def
@@ -2176,40 +2200,47 @@ void LinkerDriver::linkerMain(ArrayRef<const char *> argsArr) {
// that from entry point name. Must happen before /entry handling,
// and after the early return when just writing an import library.
if (config->subsystem == IMAGE_SUBSYSTEM_UNKNOWN) {
+ llvm::TimeTraceScope timeScope("Infer subsystem");
config->subsystem = inferSubsystem();
if (config->subsystem == IMAGE_SUBSYSTEM_UNKNOWN)
fatal("subsystem must be defined");
}
// Handle /entry and /dll
- if (auto *arg = args.getLastArg(OPT_entry)) {
- config->entry = addUndefined(mangle(arg->getValue()));
- } else if (!config->entry && !config->noEntry) {
- if (args.hasArg(OPT_dll)) {
- StringRef s = (config->machine == I386) ? "__DllMainCRTStartup at 12"
- : "_DllMainCRTStartup";
- config->entry = addUndefined(s);
- } else if (config->driverWdm) {
- // /driver:wdm implies /entry:_NtProcessStartup
- config->entry = addUndefined(mangle("_NtProcessStartup"));
- } else {
- // Windows specific -- If entry point name is not given, we need to
- // infer that from user-defined entry name.
- StringRef s = findDefaultEntry();
- if (s.empty())
- fatal("entry point must be defined");
- config->entry = addUndefined(s);
- log("Entry name inferred: " + s);
+ {
+ llvm::TimeTraceScope timeScope("Entry point");
+ if (auto *arg = args.getLastArg(OPT_entry)) {
+ config->entry = addUndefined(mangle(arg->getValue()));
+ } else if (!config->entry && !config->noEntry) {
+ if (args.hasArg(OPT_dll)) {
+ StringRef s = (config->machine == I386) ? "__DllMainCRTStartup at 12"
+ : "_DllMainCRTStartup";
+ config->entry = addUndefined(s);
+ } else if (config->driverWdm) {
+ // /driver:wdm implies /entry:_NtProcessStartup
+ config->entry = addUndefined(mangle("_NtProcessStartup"));
+ } else {
+ // Windows specific -- If entry point name is not given, we need to
+ // infer that from user-defined entry name.
+ StringRef s = findDefaultEntry();
+ if (s.empty())
+ fatal("entry point must be defined");
+ config->entry = addUndefined(s);
+ log("Entry name inferred: " + s);
+ }
}
}
// Handle /delayload
- for (auto *arg : args.filtered(OPT_delayload)) {
- config->delayLoads.insert(StringRef(arg->getValue()).lower());
- if (config->machine == I386) {
- config->delayLoadHelper = addUndefined("___delayLoadHelper2 at 8");
- } else {
- config->delayLoadHelper = addUndefined("__delayLoadHelper2");
+ {
+ llvm::TimeTraceScope timeScope("Delay load");
+ for (auto *arg : args.filtered(OPT_delayload)) {
+ config->delayLoads.insert(StringRef(arg->getValue()).lower());
+ if (config->machine == I386) {
+ config->delayLoadHelper = addUndefined("___delayLoadHelper2 at 8");
+ } else {
+ config->delayLoadHelper = addUndefined("__delayLoadHelper2");
+ }
}
}
@@ -2303,54 +2334,57 @@ void LinkerDriver::linkerMain(ArrayRef<const char *> argsArr) {
// This code may add new undefined symbols to the link, which may enqueue more
// symbol resolution tasks, so we need to continue executing tasks until we
// converge.
- do {
- // Windows specific -- if entry point is not found,
- // search for its mangled names.
- if (config->entry)
- mangleMaybe(config->entry);
-
- // Windows specific -- Make sure we resolve all dllexported symbols.
- for (Export &e : config->exports) {
- if (!e.forwardTo.empty())
- continue;
- e.sym = addUndefined(e.name);
- if (e.source != ExportSource::Directives)
- e.symbolName = mangleMaybe(e.sym);
- }
+ {
+ llvm::TimeTraceScope timeScope("Add unresolved symbols");
+ do {
+ // Windows specific -- if entry point is not found,
+ // search for its mangled names.
+ if (config->entry)
+ mangleMaybe(config->entry);
+
+ // Windows specific -- Make sure we resolve all dllexported symbols.
+ for (Export &e : config->exports) {
+ if (!e.forwardTo.empty())
+ continue;
+ e.sym = addUndefined(e.name);
+ if (e.source != ExportSource::Directives)
+ e.symbolName = mangleMaybe(e.sym);
+ }
- // Add weak aliases. Weak aliases is a mechanism to give remaining
- // undefined symbols final chance to be resolved successfully.
- for (auto pair : config->alternateNames) {
- StringRef from = pair.first;
- StringRef to = pair.second;
- Symbol *sym = ctx.symtab.find(from);
- if (!sym)
- continue;
- if (auto *u = dyn_cast<Undefined>(sym))
- if (!u->weakAlias)
- u->weakAlias = ctx.symtab.addUndefined(to);
- }
+ // Add weak aliases. Weak aliases is a mechanism to give remaining
+ // undefined symbols final chance to be resolved successfully.
+ for (auto pair : config->alternateNames) {
+ StringRef from = pair.first;
+ StringRef to = pair.second;
+ Symbol *sym = ctx.symtab.find(from);
+ if (!sym)
+ continue;
+ if (auto *u = dyn_cast<Undefined>(sym))
+ if (!u->weakAlias)
+ u->weakAlias = ctx.symtab.addUndefined(to);
+ }
- // If any inputs are bitcode files, the LTO code generator may create
- // references to library functions that are not explicit in the bitcode
- // file's symbol table. If any of those library functions are defined in a
- // bitcode file in an archive member, we need to arrange to use LTO to
- // compile those archive members by adding them to the link beforehand.
- if (!ctx.bitcodeFileInstances.empty())
- for (auto *s : lto::LTO::getRuntimeLibcallSymbols())
- ctx.symtab.addLibcall(s);
-
- // Windows specific -- if __load_config_used can be resolved, resolve it.
- if (ctx.symtab.findUnderscore("_load_config_used"))
- addUndefined(mangle("_load_config_used"));
-
- if (args.hasArg(OPT_include_optional)) {
- // Handle /includeoptional
- for (auto *arg : args.filtered(OPT_include_optional))
- if (isa_and_nonnull<LazyArchive>(ctx.symtab.find(arg->getValue())))
- addUndefined(arg->getValue());
- }
- } while (run());
+ // If any inputs are bitcode files, the LTO code generator may create
+ // references to library functions that are not explicit in the bitcode
+ // file's symbol table. If any of those library functions are defined in a
+ // bitcode file in an archive member, we need to arrange to use LTO to
+ // compile those archive members by adding them to the link beforehand.
+ if (!ctx.bitcodeFileInstances.empty())
+ for (auto *s : lto::LTO::getRuntimeLibcallSymbols())
+ ctx.symtab.addLibcall(s);
+
+ // Windows specific -- if __load_config_used can be resolved, resolve it.
+ if (ctx.symtab.findUnderscore("_load_config_used"))
+ addUndefined(mangle("_load_config_used"));
+
+ if (args.hasArg(OPT_include_optional)) {
+ // Handle /includeoptional
+ for (auto *arg : args.filtered(OPT_include_optional))
+ if (isa_and_nonnull<LazyArchive>(ctx.symtab.find(arg->getValue())))
+ addUndefined(arg->getValue());
+ }
+ } while (run());
+ }
// Create wrapped symbols for -wrap option.
std::vector<WrappedSymbol> wrapped = addWrappedSymbols(ctx, args);
@@ -2447,6 +2481,7 @@ void LinkerDriver::linkerMain(ArrayRef<const char *> argsArr) {
// need to create a .lib file. In MinGW mode, we only do that when the
// -implib option is given explicitly, for compatibility with GNU ld.
if (!config->exports.empty() || config->dll) {
+ llvm::TimeTraceScope timeScope("Create .lib exports");
fixupExports();
if (!config->noimplib && (!config->mingw || !config->implib.empty()))
createImportLibrary(/*asLib=*/false);
@@ -2500,6 +2535,7 @@ void LinkerDriver::linkerMain(ArrayRef<const char *> argsArr) {
// Handle /call-graph-ordering-file and /call-graph-profile-sort (default on).
if (config->callGraphProfileSort) {
+ llvm::TimeTraceScope timeScope("Call graph");
if (auto *arg = args.getLastArg(OPT_call_graph_ordering_file)) {
parseCallGraphFile(arg->getValue());
}
@@ -2548,6 +2584,12 @@ void LinkerDriver::linkerMain(ArrayRef<const char *> argsArr) {
rootTimer.stop();
if (config->showTiming)
ctx.rootTimer.print();
+
+ if (config->timeTraceEnabled) {
+ checkError(timeTraceProfilerWrite(
+ args.getLastArgValue(OPT_time_trace_eq).str(), config->outputFile));
+ timeTraceProfilerCleanup();
+ }
}
} // namespace lld::coff
diff --git a/lld/COFF/DriverUtils.cpp b/lld/COFF/DriverUtils.cpp
index fa15f816fb5fcd4..583f6af070b6258 100644
--- a/lld/COFF/DriverUtils.cpp
+++ b/lld/COFF/DriverUtils.cpp
@@ -31,6 +31,7 @@
#include "llvm/Support/MathExtras.h"
#include "llvm/Support/Process.h"
#include "llvm/Support/Program.h"
+#include "llvm/Support/TimeProfiler.h"
#include "llvm/Support/raw_ostream.h"
#include "llvm/WindowsManifest/WindowsManifestMerger.h"
#include <limits>
@@ -661,6 +662,7 @@ static StringRef exportSourceName(ExportSource s) {
// Performs error checking on all /export arguments.
// It also sets ordinals.
void LinkerDriver::fixupExports() {
+ llvm::TimeTraceScope timeScope("Fixup exports");
// Symbol ordinals must be unique.
std::set<uint16_t> ords;
for (Export &e : ctx.config.exports) {
diff --git a/lld/COFF/ICF.cpp b/lld/COFF/ICF.cpp
index 37f5e7549b7fc09..0f43da0dbc101af 100644
--- a/lld/COFF/ICF.cpp
+++ b/lld/COFF/ICF.cpp
@@ -26,6 +26,7 @@
#include "llvm/ADT/Hashing.h"
#include "llvm/Support/Debug.h"
#include "llvm/Support/Parallel.h"
+#include "llvm/Support/TimeProfiler.h"
#include "llvm/Support/raw_ostream.h"
#include "llvm/Support/xxhash.h"
#include <algorithm>
@@ -246,6 +247,7 @@ void ICF::forEachClass(std::function<void(size_t, size_t)> fn) {
// Two sections are considered the same if their section headers,
// contents and relocations are all the same.
void ICF::run() {
+ llvm::TimeTraceScope timeScope("ICF");
ScopedTimer t(ctx.icfTimer);
// Collect only mergeable sections and group by hash value.
diff --git a/lld/COFF/LLDMapFile.cpp b/lld/COFF/LLDMapFile.cpp
index c14480aaf821af7..58098cf5d6528d0 100644
--- a/lld/COFF/LLDMapFile.cpp
+++ b/lld/COFF/LLDMapFile.cpp
@@ -25,6 +25,7 @@
#include "Writer.h"
#include "lld/Common/ErrorHandler.h"
#include "llvm/Support/Parallel.h"
+#include "llvm/Support/TimeProfiler.h"
#include "llvm/Support/raw_ostream.h"
using namespace llvm;
@@ -92,6 +93,7 @@ void lld::coff::writeLLDMapFile(const COFFLinkerContext &ctx) {
if (ctx.config.lldmapFile.empty())
return;
+ llvm::TimeTraceScope timeScope(".lldmap file");
std::error_code ec;
raw_fd_ostream os(ctx.config.lldmapFile, ec, sys::fs::OF_None);
if (ec)
diff --git a/lld/COFF/LTO.cpp b/lld/COFF/LTO.cpp
index 09d722849c38270..7df931911213672 100644
--- a/lld/COFF/LTO.cpp
+++ b/lld/COFF/LTO.cpp
@@ -86,6 +86,8 @@ lto::Config BitcodeCompiler::createConfig() {
c.CSIRProfile = std::string(ctx.config.ltoCSProfileFile);
c.RunCSIRInstr = ctx.config.ltoCSProfileGenerate;
c.PGOWarnMismatch = ctx.config.ltoPGOWarnMismatch;
+ c.TimeTraceEnabled = ctx.config.timeTraceEnabled;
+ c.TimeTraceGranularity = ctx.config.timeTraceGranularity;
if (ctx.config.emit == EmitKind::LLVM) {
c.PostInternalizeModuleHook = [this](size_t task, const Module &m) {
diff --git a/lld/COFF/MapFile.cpp b/lld/COFF/MapFile.cpp
index f7a4ef9612907d6..ed521dd375ed010 100644
--- a/lld/COFF/MapFile.cpp
+++ b/lld/COFF/MapFile.cpp
@@ -36,6 +36,7 @@
#include "lld/Common/Timer.h"
#include "llvm/Support/Parallel.h"
#include "llvm/Support/Path.h"
+#include "llvm/Support/TimeProfiler.h"
#include "llvm/Support/raw_ostream.h"
using namespace llvm;
@@ -203,6 +204,7 @@ void lld::coff::writeMapFile(COFFLinkerContext &ctx) {
if (ctx.config.mapFile.empty())
return;
+ llvm::TimeTraceScope timeScope("Map file");
std::error_code ec;
raw_fd_ostream os(ctx.config.mapFile, ec, sys::fs::OF_None);
if (ec)
diff --git a/lld/COFF/MarkLive.cpp b/lld/COFF/MarkLive.cpp
index ad8c340f1845177..2cf216a6aaad564 100644
--- a/lld/COFF/MarkLive.cpp
+++ b/lld/COFF/MarkLive.cpp
@@ -11,6 +11,7 @@
#include "Symbols.h"
#include "lld/Common/Timer.h"
#include "llvm/ADT/STLExtras.h"
+#include "llvm/Support/TimeProfiler.h"
#include <vector>
namespace lld::coff {
@@ -19,6 +20,7 @@ namespace lld::coff {
// COMDAT chunks will be ignored by Writer, so they will be excluded
// from the final output.
void markLive(COFFLinkerContext &ctx) {
+ llvm::TimeTraceScope timeScope("Mark live");
ScopedTimer t(ctx.gcTimer);
// We build up a worklist of sections which have been marked as live. We only
diff --git a/lld/COFF/MinGW.cpp b/lld/COFF/MinGW.cpp
index 53e146bb8600b8f..e46f5277a8c3654 100644
--- a/lld/COFF/MinGW.cpp
+++ b/lld/COFF/MinGW.cpp
@@ -16,6 +16,7 @@
#include "llvm/Object/COFF.h"
#include "llvm/Support/Parallel.h"
#include "llvm/Support/Path.h"
+#include "llvm/Support/TimeProfiler.h"
#include "llvm/Support/raw_ostream.h"
using namespace llvm;
@@ -172,6 +173,7 @@ bool AutoExporter::shouldExport(Defined *sym) const {
void lld::coff::writeDefFile(StringRef name,
const std::vector<Export> &exports) {
+ llvm::TimeTraceScope timeScope("Write .def file");
std::error_code ec;
raw_fd_ostream os(name, ec, sys::fs::OF_None);
if (ec)
dif...
[truncated]
``````````
</details>
https://github.com/llvm/llvm-project/pull/68236
More information about the llvm-commits
mailing list