<table border="1" cellspacing="0" cellpadding="8">
<tr>
<th>Issue</th>
<td>
<a href=https://github.com/llvm/llvm-project/issues/107819>107819</a>
</td>
</tr>
<tr>
<th>Summary</th>
<td>
[Clang] -O3 don't generate branch instruction
</td>
</tr>
<tr>
<th>Labels</th>
<td>
clang
</td>
</tr>
<tr>
<th>Assignees</th>
<td>
</td>
</tr>
<tr>
<th>Reporter</th>
<td>
wooffie
</td>
</tr>
</table>
<pre>
Using -O3 optimization removes branch instruction and program gets segfault
```
clang version 18.1.8
Target: x86_64-pc-linux-gnu
Thread model: posix
InstalledDir: /usr/bin
Found candidate GCC installation: /usr/bin/../lib/gcc/x86_64-pc-linux-gnu/14.2.1
Found candidate GCC installation: /usr/bin/../lib64/gcc/x86_64-pc-linux-gnu/14.2.1
Selected GCC installation: /usr/bin/../lib64/gcc/x86_64-pc-linux-gnu/14.2.1
Candidate multilib: .;@m64
Candidate multilib: 32;@m32
Selected multilib: .;@m64
```
Problem occurs in ffmpeg project
Expand sections to see details about compilation
<details>
<summary>FFmpeg version/configure</summary>
git clone https://git.ffmpeg.org/ffmpeg.git --branch n4.4-dev --single-branch --depth=1
```
./ffmpeg/configure
--prefix=.
--enable-shared
--disable-static
--enable-pic
--disable-asm
--cc=clang
--cxx=clang++
--ld=clang++
--disable-stripping
--disable-inline-asm
--enable-version3
--enable-swscale
--enable-v4l2-m2m
--disable-outdev=fbdev
--disable-indev=fbdev
--disable-openssl
--disable-xmm-clobber-test
--disable-lto
--enable-debug=3
--disable-programs
--disable-postproc
--disable-doc
--disable-htmlpages
--disable-manpages
--disable-podpages
--disable-txtpages
--disable-sndio
--disable-schannel
--disable-securetransport
--disable-xlib
--disable-cuda
--disable-cuvid
--disable-nvenc
--disable-vaapi
--disable-vdpau
--disable-videotoolbox
--disable-audiotoolbox
--disable-appkit
--disable-alsa
--disable-cuda
--disable-cuvid
--disable-nvenc
--disable-vaapi
--disable-vdpau
--disable-sdl2
```
</details>
<details>
<summary>Compile options stored in ffbuild/config.mak - O3 optimization</summary>
```
CPPFLAGS= -D_ISOC99_SOURCE -D_FILE_OFFSET_BITS=64 -D_LARGEFILE_SOURCE -D_POSIX_C_SOURCE=200112 -D_XOPEN_SOURCE=600 -DPIC -DZLIB_CONST
CFLAGS= -std=c11 -fomit-frame-pointer -fPIC -pthread -g3 -Wdeclaration-after-statement -Wall -Wdisabled-optimization -Wpointer-arith -Wredundant-decls -Wwrite-strings -Wtype-limits -Wundef -Wmissing-prototypes -Wno-pointer-to-int-cast -Wstrict-prototypes -Wempty-body -Wno-parentheses -Wno-switch -Wno-format-zero-length -Wno-pointer-sign -Wno-unused-const-variable -Wno-bool-operation -Wno-char-subscripts -O3 -fno-math-errno -fno-signed-zeros -mstack-alignment=16 -Qunused-arguments -Werror=implicit-function-declaration -Werror=missing-prototypes -Werror=return-type
CXXFLAGS= -D__STDC_CONSTANT_MACROS -std=c++11
```
</details>
Code fragment (ff_seek_frame_binary libavformat/utils.c:2155):
```
index = av_index_search_timestamp(st, target_ts,
flags & ~AVSEEK_FLAG_BACKWARD);
printf("index = %d\n", index);
printf("st->nb_index_entries = %d\n", st->nb_index_entries);
//expanded av_assert0(index < st->nb_index_entries);
do {
if (!(index < st->nb_index_entries)) {
av_log(NULL, AV_LOG_PANIC, "Assertion %s failed at %s:%d\n",
AV_STRINGIFY(index < st->nb_index_entries), __FILE__, __LINE__);
abort();
}
} while (0);
if (index >= 0)
printf("branch inside\n");
e = &st->index_entries[index]; // SEGFAULT
av_assert1(e->timestamp >= target_ts);
pos_max = e->pos;
ts_max = e->timestamp;
pos_limit = pos_max - e->min_distance;
av_log(s, AV_LOG_TRACE, "using cached pos_max=0x%"PRIx64" pos_limit=0x%"PRIx64
" dts_max=%s\n", pos_max, pos_limit, av_ts2str(ts_max));
}
```
Index is local variable int type
Code output:
```
index = -1
st->nb_index_entries = 1
branch inside
```
Expected two branch instruction - for `index < st->nb_index_entries` and `index >= 0` but got only one:

`jge` to `ff_seek_frame_binary` is assert code. This part in disassembler:

Behavior with -O0 (manually changed in config.mak, another options same):
Code otput:
```
index = -1
st->nb_index_entries = 1
```
Asm:
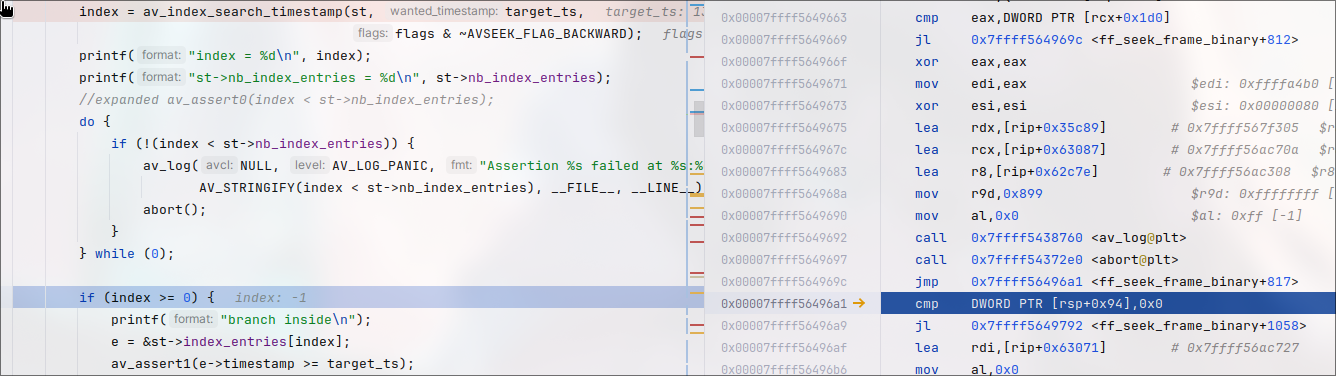

Compiler with -O3 optimized out needed branch =)
</pre>
<img width="1px" height="1px" alt="" src="http://email.email.llvm.org/o/eJy8WFtz6ygS_jXkhcIlI1_khzw4vpxKTTbJJjmTs_uiQqIlMweBCpDjzMP-9i2QfInlnJmpnbOulGO6m77x0TQwa0WpAK7R-AaNl1escRttrt-0LgoBV5nm79coWqJo_tUKVWLyEGNdO1GJ35kTWmEDld6CxZlhKt9goawzTR5YTHFcG10aVuESnMUWyoI10rUKu-9J1P2FYS6ZKvEWjPUahslgOEhazgszJTgUz_EumaSTEalzIoVqdqRUTSeyMcA4rjQH6QVrbcWuZd0q65iUwJfCeBai68YaRNeZUK3EWjeK45wpLjhzgL8sFiEaJmWItDeLrgcDRNdSZIiuyzxHdH3JM7oejgZ0MPyfrUxGf8HOM0jIHfCfZmBxCKFqpBM-C_EcD1B8g0ZRNRn9SCqmnVhMz7z9ka4zpLTfj0ZnEiqs87wxFguFi6KqofTA-w3yD1Bb7WoPSQsBnhY7jS0A5uCYkBazTDcO57qqRZeqdm686CRQvGpJGKN4YZuqYuYdxav1OljsQIvoOteqEGVjAMULRNdHycP0D__3o1I4nEutAG-cqy2K54iu_ZIIN2ijGmhTIrruBl6ekG7jqdFgRDhsMSF-o0rYMwjhULsNipfDM7Mf8zk4KD6NoJMlpDZQiB2Kl4MDCRTLJBC7YQb4gcqFbcmOOZGfC9cnpL0ks9WBlucoXrZF4EDa7fY0RG8QvTlwJP-EcfTBiLoWJ8r2HKGkUB9Ndx52yxj3wnyzOZPQEx9JSipa9SzoxnHYonhZZH5Z-g78iKtrUNbKHn1XVSSXOsvAEAfW9QSk0-cecsiaEsXLuCfcFWfbZ2jraqPzczrX_dXbuErWrIS-loqpy4xa88sMt3OXGVZxoXvEfMOUgn6WLOSNAWeYsrU2_STtpMh6xLzh7AJxK_rQVltQ_URsGatFn8pr1vSpgoN2WstM7_r7oeHic2Zdfxf9kJi0l7z_P4ZkuaSXC3Uoguc19PPi-qG2LkI5htBz-JptnfbFJtT5rBGSH4rVoGLfMcFn_cknFfiio4vHx_Xd_MszipeYLNPb54fFbJY-P3x9Wqw8YX17t0of1uvn1Ut6c_vi5SYjz7ibP31ZBe5R-PHh-fZbuugoKF7SKBoOqWd9e3hc3R8ZkyjCZPl4u8Bk-e-725t08XD__HKapcXBLYyJdaHqDYeYFLoSjhSGVX5PCeXAYFIETbVre6HwIWWMySuHXDITskJY4cCEEg0VKIfJK5PSy7SLycmHHo-8dtoJM8JtMHk1wBvFmXLEa7WYvL4Z4dqCq0o_du81ECkq4fyoURwKTF4rYf3p5CuP017EM5Xee0-cJkI5kjPrffLacvdRGKravRPfl3YzmQHlNmD3quybcP7Y878LbSrmyO9gNJGgSrf5aM73vi2lUY0FTnKtrCNbZoRPQ8vKtJa-IJt9MpQm-YYZYpvM5kbUPsKHGJNCaVIxtyFgjNLtOHTXPHhgMamsY_l3wqQolc-7P5QnmPyzs85M2XhyiNMYbVC8FFUtRe6XuVGhbyEn63gidzGze6YB1xhFPPkDrr59OyKLLNP0-WW5aOE3v39J_zFfPD08HxDXnrHD4V_e5AvNAReGlQFqiCZFkVqA72lAbpoJxcw7liJj23bBfH_qhLSDHMVzOhyPEZ35buiSYdx9_Hm6wz4Stk3DILXATL5JnajAOlbViCbWIbrALlwlUmcRXXRaCslKixGd4P_Mf31erX5JfWbSm_nil9f50zI4cPPRYm2EcgWiCaL0aB3RMUfjhUKUelOB8UezrSMoXqms8xuUM8Ifgn11lyUv6W9bRwgtL3CfFGYtGBchmuy9_Sv6uMZoekYLeS9wCGL4J_XS2WU9_sO2qdQlosn917s7H-781_Tu4Uv6OL-_XfgxonQeovDYR3RsccGE9OG5MAwt82nCOjvzX9Pnl6fb-y-363_9WT8XOG0rftr-vru9D79PUsMybVyIvp-vsAbT5dmiTJf4bePPM0ST6HTaGZhDUvdu-kMCB-mehVMUHW_ggsMhBZc9gw5ckzYBH6Mf37SoHS9RfNMBCT-vvqznX-9e-roO0PIQAK_usOP2zp9suMv-1NqmFWt3UFBRa3tR0J3JHTf3Z2rDGRQm7I2QdmolVMqFdUzlcLKmewzaEwC-PM0Xqw6ATXgHyVm-Ab5XieJltEN0jCh9fLrd-Ys0PRrvcy-i_9LH6-FubyNA_FgM9sa7n60tuvAhOEutM4gm3dyw8Y5BHoB58U59G3AnLJY6ZxIfTkOhHO4dIb6268bVjfusQh9rI-nOjh_Uu07iI5Y_d3W1q9uHA_emLz1BEVxog9Ek-sMtP4nCe9WJaLftJhHOGodL7bBW8h1rBcdI22869HumYiX4PUOT3vV902SDXFfh3cV3Uc6xfBNOekTXfvOEHzkUwPMZEBYVYzKiU05mMzYivKCjEWWjJJnlhypwSMpvJXgf_b1vEl06WT1XWNzuUZxrDgP8shEW18w430n7ps9aqDIJ5ieFNk6K2YTOJmQyohMymhRjMhtFCRnmLGNxMcrYdHoW2g1s2FZog99Cy_kQ-ZJYMdUwKd-xv_qV7UXg2P4H8CvtNmCOFwZWwYf24QS2fzdqz1TMbfWT0sln4yznkJFhlI3JCKYxyRhEBOiMDZOomMbFeTr_NpBOE554g9MZy8hoOpoSVhRA4gR4kjHIh5Pi3HSX8nCTO6zn4Z4G3BcQrAB8o9Jt4lDtLmjpf1_x65jP4hm7guvhlI6TSTIdxleb61nMGUviCWdDxmmS8DyGIp-MprPpeJgn8ZW4phEdRbNoFk3HSRwPaJ4kMz4dTWCazCBP0CiCigk5kHJbDbQpr4S1DVwPo2kynF1JloG04eWc0u4ZiqLx8spc-wkka0qLRpEU1tmjCiecDM_tizBjvAy54FohOnW4BOUvGnChmF01Rl7_YNW8he4f2b9-0nXw2C9c5_T2mv43AAD__9UsLm8">