<table border="1" cellspacing="0" cellpadding="8">
<tr>
<th>Issue</th>
<td>
<a href=https://github.com/llvm/llvm-project/issues/60024>60024</a>
</td>
</tr>
<tr>
<th>Summary</th>
<td>
`swift_error(nonnull_error)` attribute in a protocol method with scalar return type and `NSError **` parameters imposes mutually exclusive requirements
</td>
</tr>
<tr>
<th>Labels</th>
<td>
new issue
</td>
</tr>
<tr>
<th>Assignees</th>
<td>
</td>
</tr>
<tr>
<th>Reporter</th>
<td>
TheDreamsWind
</td>
</tr>
</table>
<pre>
Consider the following Objective-C protocol exposed to Swift codebase:
```
@protocol TDWFailable
- (NSInteger)failableMethod:(NSError **_Nullable)error __attribute__((swift_error(nonnull_error)));
@end
```
Since `nonnull_error` should preserve the return type, when trying to conform to the protocol in Swift, it requires it to have both `throw` and "bridged" `Int` return type:
```
class SwiftDelegate: TDWFailable {
func failableMethod() throws -> Int {
return 0;
}
}
```
This, however, is not possible to compile, because of the following error:
> Throwing method cannot be an implementation of an @objc requirement because it returns a value of type `Int`; return `Void` or a type that bridges to an Objective-C class
At the same time you cannot remove `Int` return type or replace it with `NSNumber` as it causes another error to appear:
> Type 'SwiftDelegate' does not conform to protocol `TDWFailable`
Long story short - the errors are mutually exclusive, and impose conflicting requirements to the implementation. The same set of attributes for a Objective-C class, however, compiles and works without issues:
```
@interface TDWObject : NSObject
- (NSInteger)failableMethod:(NSError **_Nullable)error __attribute__((swift_error(nonnull_error)));
@end
```
In Swift it even exposes the same signature with `throws` and `Int` return type at the same time:
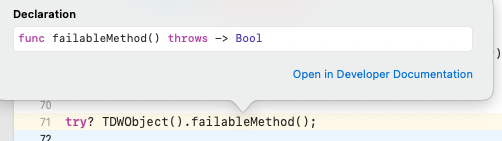
During runtime, however, this method is not reachable, and it causes unrecognized selector crash:
> Thread 1: "`-[TDWObject failableMethod:]`: unrecognized selector sent to instance `0x60000000c080`"
Assuming `swift_error(nonnull_error)` attribute is viable only with reference types, I think this should be explicitly given in the documentation, or even cause compile-time error when the attribute is applied to methods with scalar return type.
</pre>
<img width="1px" height="1px" alt="" src="http://email.email.llvm.org/o/eJzUVl1v4roW_TXmZSvIcb7ggYe2TKVKd3qv1OrOY-U4G-IZx861HRjurz-yHSh0OufoPB6EIMGxvfZaay_DnZN7jbgh1T2ptgs--d7YzWuPW4t8cN-k7hat6U6bB6Od7NCC7xF2RilzlHoP_26_o_DygNkDjNZ4I4wC_Dkahx14Ay9HufMgTIctd0iKO0K3hN6Rms7vdFvSy-TX7bdHLhVvFabBDAhbPb88aY97tIStd_PwV_S96cKaYfyLtcYCYXeE3b09TyqtwNYYf397495b2U4e394IWxG2cgHaWxwmbKWN1pNS5_v1_C7uLwhRd5-Cf5FaIJCa3i5RU3C9mVQHo0WH9oCROot-shr8aUTCHuDYowZvT4FLb0AYvTN2CJfh4QspUicmwxTpweL_JmnRhWtvoOcHhNb4PqDwvTXHsDvXHRDGWiu7PXaEsTD6pH0Yu0bxG02E4s6lXbeocM99ePRaHiDNzA4AwG7SAj5IE3heQ0TkICPFF3jS_nZaeM1o6IVtIM12BnW5uEX32ksXyOjNEQ_BFQ8gHWjjYTTOyYAu0jmMUkWiWxR8cghm98HBSa0LCcUXeA14w9AQqwDBdVi4ReAa5DAqHFB77qXRYTmugZTUtN_FWZcwfNkwyhXqc8DhwNWUMJxGfNeDFPdnEkhN_2tkF0QyFnh60PfcQxLShbq4vmm8KNWMP37e-Vik4wOClwPCyUznMiwO5oCfeyFsaXFUXETcR5ks9fzyPA0tRk_zaLpYmgOuje_RJg4jsHFE_oHNWClrbp3EGugMJsWuTH8xPKnpdRBcgiJ-_svoPThv7Cm0mPWQxXIjCgfcIgyTn7hSJ8CfQk1OHqIHQkfIIWRT3FNJ4YPMV6q5c-fdyryE1zOdDn0U_ZwmDnZRp1_1uHXnbEUXQRyN_eEivWbyIJ2b0P1JNkrt0e6CKK_bb2kjCL34_JJu_hk5-TRnWPAPHlDPx4R7t2o4i7ifLF6cl6LjkmafepZ_MPs7kSwn1f2LsIja9cYDo6zIaJ7lZZiUQ8GgqOA_X0m1JWzVez9GFdgjYY-TQ5vJge_RLffS91MbfhFGe9R-KcxA2GPOVnlBG0bYI8tZWTcrus44a7pm3ZVZyxuRlU1Hs1VZsawWFcVq1QlRiuWo94G1K09vJxu9OOlYw615fC_dOY3mmLPIRZ-km4196cpJWxRmr-X_sQOHCoU3FoTlrv-Yc8g7yIOVCGOkphmp7t8t9qt_qm0Mq7vfbOFC7nkDUjvP50OR_qxpegm6imZg7CaqnJuGUDmp6V84LbjgbM5Aw0HGU8hodUqGsbhDi2HjYIzYgU-BO_0jMTifxy0G6ykppFcn2MvgRamjiTojpkvXh_nGJq-mLJ-bOIuZmhomHeA93kLj46hk-geUZEvdDk5wxe21fZeLblN062LNF7jJ66ao16wuikW_aVgpilJUbbvaVRS7okNaVDlj1Y6WImcLuQmGpnle5rRo8mK5o6uiqxpeVrToWEVDMw5cqqVSh2Fp7H4Ro2ZTU8rKheItKhf_-TGm8ZhyKMhTbRd2E-Zk7bR3pKRKOu_eV_HSK9z8XcE08Pd8n838G1LO3X6bVmG9kVs-oEfr5iR3n2T9TaIvJqs2t72d-nlu4lDV_JWN1sQ8ZY9zJrPHyNUfAQAA___H-6V3">