<table border="1" cellspacing="0" cellpadding="8">
<tr>
<th>Issue</th>
<td>
<a href=https://github.com/llvm/llvm-project/issues/56718>56718</a>
</td>
</tr>
<tr>
<th>Summary</th>
<td>
[Stack protector] Unnecessary stack cookie checking
</td>
</tr>
<tr>
<th>Labels</th>
<td>
new issue
</td>
</tr>
<tr>
<th>Assignees</th>
<td>
</td>
</tr>
<tr>
<th>Reporter</th>
<td>
JianxiaoLuIntel
</td>
</tr>
</table>
<pre>
The flag **-fstack-protector** inserts a guard variable (aka stack cookie) onto the stack frame for each vulnerable function. For example, if a function will write a buffer, after the buffer is written, the stack cookie need to be checked to make sure the buffer overflow didn't happen.
Consider the following test case:
```C++
#include <cstring>
#include <cstdlib>
#include <cstdio>
#pragma warning(disable : 4996) // for strcpy use
// Vulnerable function
__attribute__((noinline)) int vulnerable(const char* str , int a) {
if (a % 2 == 0)
{
// Write buffer
char buffer[10];
strcpy(buffer, str);
return buffer[0];
}
return 0;
}
int main() {
// declare buffer that is bigger than expected
const char* str = "This string is longer than 10 characters!!";
printf("%d\n", vulnerable(str, std::rand()));
}
```
The function is vulnerable because it may write the buffer, so there will be a stack cookie on the stack frame. If it enter the branch which write the buffer, the stack cookie need to be checked to avoid buffer overflow.
**If it enter another branch which won't write any buffer, ideally, it's unnecessary to check the cookie. However, we found it will be checked in fact.**
Here is the assembly code:
On Windows:
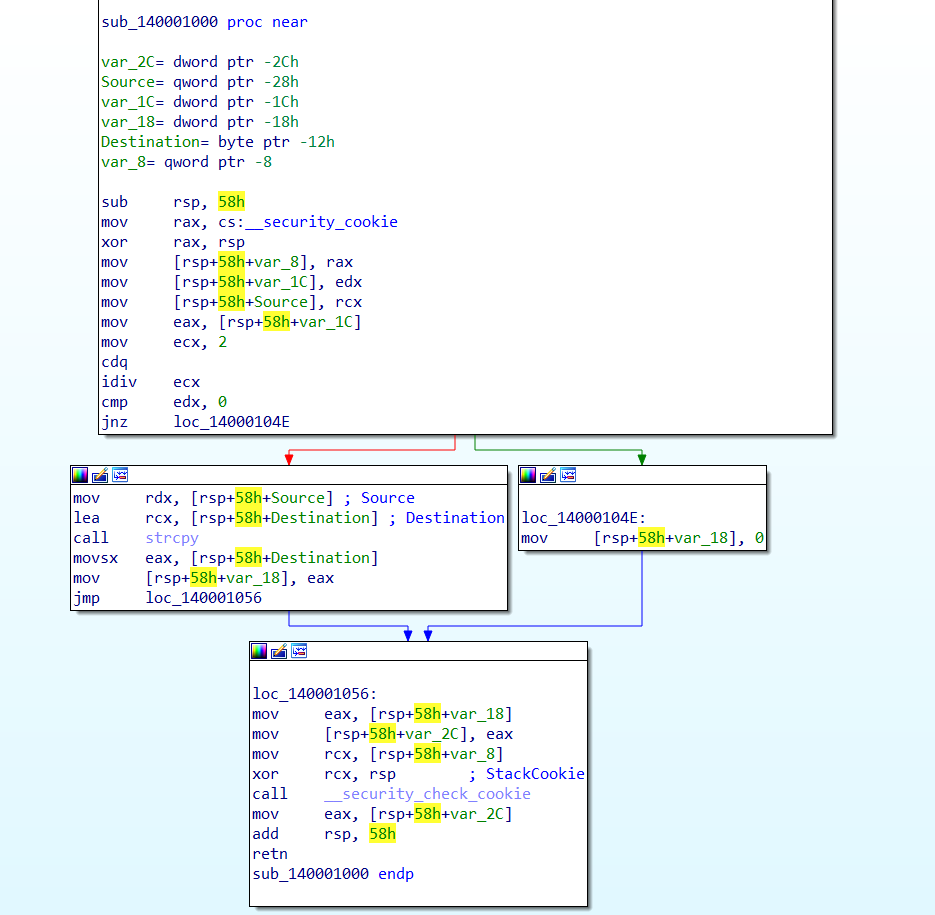
On Ubuntu:
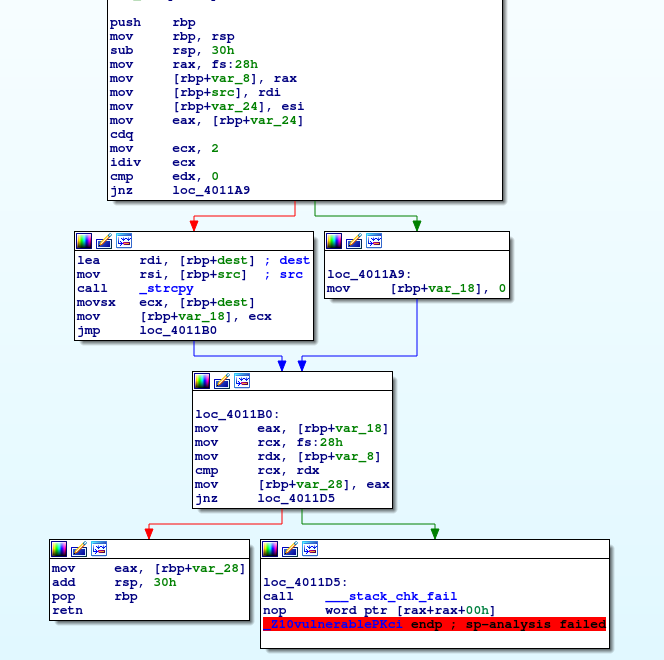
**By the way, I wonder whether we can inline the __security_check_cookie on Windows.**
</pre>
<img width="1px" height="1px" alt="" src="http://email.email.llvm.org/o/eJytVk1v4zYQ_TXyhZAgUd8HHxKnwW5RoIfudo8GJVISG5o0SMpa__sOKdmSk7TooQEhy5rhzNObxxc3il733waGOkF6FOAnWGFnLGnfwrNWlrVW6fkx4tIwbQ0iqB-JpuhCNCeNYLCtIm8E-V2oVeqNswDXSEmrkIXac6DT5AR9lEaMtAO6jEIy7fd3o2wtVzJC6NWFf5LTWUCJA-IddLuF0cSFQJPmlsHTZuw6pl0S6SzTvtH8DHHjsyyTLrwimKEhyRhFAK1hqB1Y-zZ_O5E3yBs121ZSF6Y7oSZEOYVipUUDOZ-ZjIL4JYif5utBScPpAqFTAvK57JFlxqKWGBakS2JQxPM6BPjZrfkpTrlsxUiByPTQGqthd5D-8g9RKnjzL1GutsGzJv2JoIlo6YriinIzjyx9QlldF25OCAb4CsvPBtq35ysaAfbmFZeEPz_ObI4fj8QC7ma07HiENrCk4lJw6ZTgmnBpNyOHeAusAT8DcfJybZEfOKQRlx-UN3riGlTgJAaXHGGA_gILxa7wLeOejd79LcB_eNEskrlvimvX_vY4f07iIIfaz9uMmQ9ov-oNHrnej3ma2VHLtdaHUuXL_X7Jjdf4GnRXR8KJcOmJfKRieR_KWkH0XaZ2INapvuF9P3-VcIrOcHgZvW_9hHCgMcD42wBbZ9m5IkLJe5Ek9hsIFNImwIlfePteZ9hmO48Uw3xokB-kvz08jtuT5rij7jikT5pIOr_fsj5ycTstW2q8Vd38AMBuXKRhLQHZIu7Iuy4-sZ5l3937EfDmnaRxNvLgDFDznV9F6GvnKjJ59xhADvY1DdxdP2nyH_2GXBSn733m5ivecLediVQO-bvuavakxRLldYMCDIkIcfW3FrIMGqVkLTOG6Kvr76F4sDPMCH1RE7vMuydnZKOkDsCNqxt2LlEHgohmkNvhfHHUwlBcUWIMOzXiCtXp6oC_S_SDS6oms5oiSCp_BjnLHmzTnRpcDdaefYZXOwxVh_xEemaintthbNwTkDMYvI1adYKcKkuSsihTuE2quE7yJMZhQVtS4JiGaZmkYZYVNCRlnoQ4o02S5W1cNl10dr5Yb18DQH5vRmnHzzCOc-R_g5nUYVGkadnUaVikeRlmBC6kbrqwyMq2rkgMSPNPYM78P1893RPxo_7qNOH-FU0D83qBQbZwjmcr9pnHo2HtCIq5Hv1Ej6v2l9F8Mtkd2ydFXlVxnsfxju5TWqc12VluBdsDNX94va8_GPIX9H0jt4fj4LuC1-xGLfaPFM60LVwJcbl9uJ8if0Fl-MqNGRlY0WtelEm1G_Y17WrMgNeqxXWTxXCb4TRJsiLOa1zVO0EaJoxDCb4k2YR8CedR-cuO73GMcVziIk6TKqkjXLKypGWDky7pWFUGUBG8WEQOR6R0v9N7D6kZewNBwY01axBkz3s48L4d1CejHZTe_8qJ_MmJ-m38CnIQOw9h71_hbxoW8Gw">