<table border="1" cellspacing="0" cellpadding="8">
<tr>
<th>Issue</th>
<td>
<a href=https://github.com/llvm/llvm-project/issues/55768>55768</a>
</td>
</tr>
<tr>
<th>Summary</th>
<td>
clang-cl: codeview debug info written by clang isn't sufficient for natvis files in C++20
</td>
</tr>
<tr>
<th>Labels</th>
<td>
new issue
</td>
</tr>
<tr>
<th>Assignees</th>
<td>
</td>
</tr>
<tr>
<th>Reporter</th>
<td>
fsb4000
</td>
</tr>
</table>
<pre>
```C++
template <class Value_type>
struct node { // list node
node* _Next; // successor node, or first element if head
node* _Prev; // predecessor node, or last element if head
node(const node&) = delete;
node& operator=(const node&) = delete;
static node* Buyheadnode(void* buf) {
node* _Result = (node*) buf;
return _Result;
}
};
int main() {
alignas(alignof(void*)) char buf[128]{};
node<int>* l = node<int>::Buyheadnode(buf);
return 0;
}
```
```
clang-cl.exe main.cpp /std:c++14 /nologo /Z7
cvdump.exe main.pdb > clang14.txt
```
```
clang-cl.exe main.cpp /std:c++20 /nologo /Z7
cvdump.exe main.pdb > clang20.txt
```
clang14.txt has
```
0x1004 : Length = 46, Leaf = 0x1505 LF_STRUCTURE
# members = 0, field list type 0x0000, FORWARD REF,
Derivation list type 0x0000, VT shape type 0x0000
Size = 0, class name = node<int>, unique name = .?AU?$node@H@@, UDT(0x00001010)
0x100f : Length = 90, Leaf = 0x1203 LF_FIELDLIST
list[0] = LF_MEMBER, public, type = 0x1005, offset = 0
member name = '_Next'
list[1] = LF_MEMBER, public, type = 0x1005, offset = 8
member name = '_Prev'
list[2] = LF_ONEMETHOD, public, VANILLA, index = 0x100A, name = 'node'
list[3] = LF_ONEMETHOD, public, VANILLA, index = 0x100C, name = 'operator='
list[4] = LF_ONEMETHOD, public, STATIC, index = 0x100E, name = 'Buyheadnode'
0x1010 : Length = 46, Leaf = 0x1505 LF_STRUCTURE
# members = 5, field list type 0x100f, CONSTRUCTOR,
Derivation list type 0x0000, VT shape type 0x0000
Size = 16, class name = node<int>, unique name = .?AU?$node@H@@, UDT(0x00001010)
```
clang20.txt has only
```
0x1004 : Length = 46, Leaf = 0x1505 LF_STRUCTURE
# members = 0, field list type 0x0000, FORWARD REF,
Derivation list type 0x0000, VT shape type 0x0000
Size = 0, class name = node<int>, unique name = .?AU?$node@H@@
```
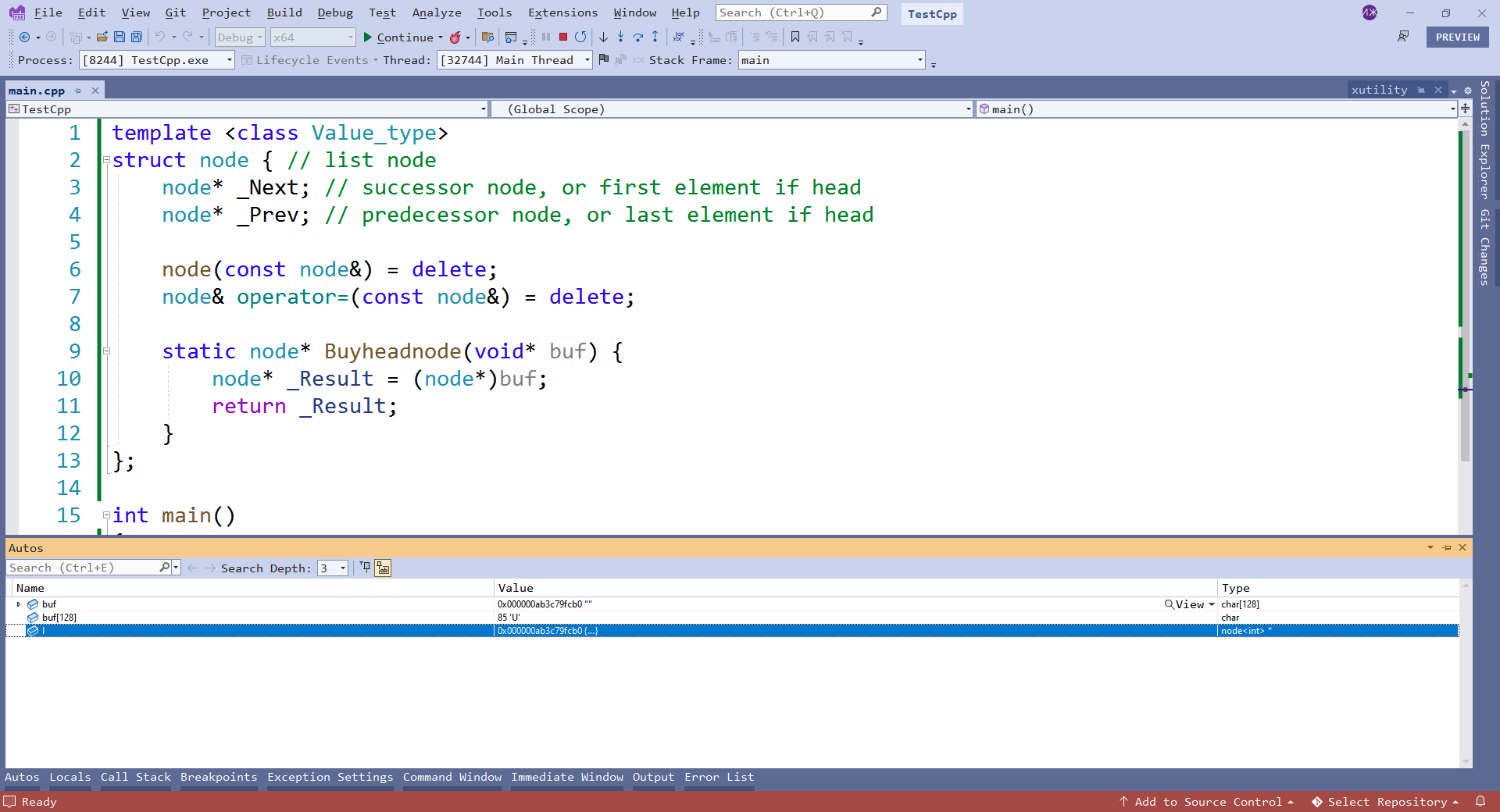
MS STL has a similar structure in `std::list` and the debugger can't visualize the `std::list` correctly in C++20 with clang-cl.
Natvis error log:
```
Natvis: C:\Program Files\Microsoft Visual Studio\2022\Preview\Common7\Packages\Debugger\Visualizers\stl.natvis(1185,31): Successfully parsed expression '_Mypair._Myval2._Mysize' in type context 'std::list<int,std::allocator<int> >'.
Natvis: C:\Program Files\Microsoft Visual Studio\2022\Preview\Common7\Packages\Debugger\Visualizers\stl.natvis(1187,57): Successfully parsed expression '_Mypair' in type context 'std::list<int,std::allocator<int> >'.
Natvis: C:\Program Files\Microsoft Visual Studio\2022\Preview\Common7\Packages\Debugger\Visualizers\stl.natvis(1189,21): Successfully parsed expression '_Mypair._Myval2._Mysize' in type context 'std::list<int,std::allocator<int> >'.
Natvis: C:\Program Files\Microsoft Visual Studio\2022\Preview\Common7\Packages\Debugger\Visualizers\stl.natvis(1190,28): Error: class "std::_List_node<int,void *>" has no member "_Next"
Error while evaluating '_Mypair._Myval2._Myhead->_Next' in the context of type 'TestCpp.exe!std::list<int,std::allocator<int>>'.
Natvis: C:\Program Files\Microsoft Visual Studio\2022\Preview\Common7\Packages\Debugger\Visualizers\stl.natvis(1184,4): Ignoring visualizer for type 'std::list<int,std::allocator<int> >' labeled as 'std::list<*>' because one or more sub-expressions was invalid.
Natvis: C:\Program Files\Microsoft Visual Studio\2022\Preview\Common7\Packages\Debugger\Visualizers\stl.natvis(1172,31): Error: identifier "_Mysize" is undefined
Error while evaluating '_Mysize' in the context of type 'TestCpp.exe!std::list<int,std::allocator<int>>'.
Natvis: C:\Program Files\Microsoft Visual Studio\2022\Preview\Common7\Packages\Debugger\Visualizers\stl.natvis(1171,4): Ignoring visualizer for type 'std::list<int,std::allocator<int> >' labeled as 'std::list<*>' because one or more sub-expressions was invalid
```
</pre>
<img width="1px" height="1px" alt="" src="http://email.email.llvm.org/o/eJztWN1v6jYU_2vCiwVynISEBx4ggG4l2l61tJP2UjmJA95CwmyHtvvrd44DBCjd_dR0t12aGsc-3z4-_pmkyl6HTp82T-ywMT504tCREetNwY0gjhenBdeaPPKiFk_mdSMcb9oQaaPq1JCyyoAuHBOHzeAhhdTNYENF4GPf2Ig83YgX43gHUl2nqdC6UjuKmEA3lwoEiEKsRWmIzMlK8OyCrI9KbI9kbZTIxFtpYPw7ws5FRmlV7i1nfYcNwPkJyYDXgM_jc_I-qTZCcVMpIPtM7laGNtzI9ODMuH5Fw3aGbCuZ4WhS51ZQeKT8JAR3QteFsZqAbTeMLMjpnXEpYWpV7plOpp1wsjMQOqfGSgjbmssS5L-xhRdyWXINU7ZX5a3tQIvk6Yora0wwdkFAMEH-Ix0Hb7wYFGFmgVuFdeh02BvBcxqlJjononYu0taHg2P7ND_27WwQEr1cdtOiJ16E9bmXbjaYXtpkoD1tNojrExwrq6JaVtj7Ndyxb7N6vWmZN1kCjkyJFev6PQOpf9GWr7GC0a-xgtH3rWiVN7aSFazsJUr64lLqg9ARmYtyaVZ2ufw-bri54Ll9BaKABmQ-e7pf3D3Ei4e76V4PJIZH1mKdCKUbWuSEbS-KrCkeWGVAAoUPTs1u734Z3U3I3XSGrwc5E6HkFrZRVV5ke1wQveIwdDy-572Xf4pWeVPiSr4WF1IP5utS_lGLlqDneLPRAzQO8y2xTz_APz5A_TBZQHo2Cl34wyw9-N6GMD8P4YCeh5BRD0M4u5rOJ_Or-8VBDPoLe4rCjrK0QHQ9vR5P71DCpk4KmWLPer6TRWlgS2Kea2F2rrdWDZoFaV10WNhUaxaea3W_SWv0Ka22rr_Vyo603t5Mr6eLD7eTU8WPo5ur-XyEXVlm4qU1wo4da2lqyBsl3rcoic-VnBwQb3T5n9Z1vxgtruILqqbnqk5KY3i8pZHepd9xuwbvbFdMaZyKb28aIbd333-7uv1_aL8OPlUlm1qKVZJUZfH6s1R-cegvBphBdRk7E-qMI9uGtp3a1nUmrhNR22_avm0D206OuAKEGixaGbPRiB0sRKy1UF255kuhe0tpVnWCI4DbDGDDXlqtgcZn0SDyQ-i5IY0GXuSybhKmUeRS1mVMDLq-CNJulPl-1-OcBkmSB4mf9Tbl8qjOt-31PeziuU0TTrRcywIQUYOcayVgXxPwvTna4bGloU8JLzNiVgLwY1Ivl1AhUw4ILDRkK3UNYAtWBKcvsaaVUiI1xSvKjg9o4RkcJgdscWzhDTcglQilEC5XSxR3aWkaOszlGEmC-KOqloqvyUwWQsP7tUxVpavckEdrJbk3dSYrmGGUMcsgtlI8Qy-u1uuqDHGMp7_jikB3snMWuo97NxVOaFP0ykY7i1w3wgrkuRb8jch9c4fI6wJc3nClRUbEC9wGtMZ0xxPl-nXDperB95YXDL81iIYZDJFNeZsEsJth7DSgTWqz-DDKi6JKm6K-y3piMz_s_WhRgiSOg_BLo_RfjwqU1Jj9zJ2_j5JFovbCh8ZMsTJgp6n9DmMHj57m4OjT0SHAYrwAErwBomfMFr6y2h1iyLtDlay9tVn55HkFThIBUa7hoCqX70QfUU4XZO_BqV2GVbsKVb7DnyxcCG3ijb0LwbHyxWvzYy5N5IPV_m5lruDSrTBWh2NBkRxiuY_A1-YjKXgiCtgMXF8Ss1_dkCQi5XCIAgIS-GPLuoIjTddJt91EmjyDEFnC-snsRwtmyI5PkkOeywwAgQSU1STsfsszAsdkDUA8l6XIPjN_j6vF_yhNQ_dfnKYX4U8nG3rZwBvwjpGmEMM9lrKFEQogRq3BayAmr8izkgaAJUleG9gFydNAOF3nuUwl_h6JQWiCBpgeFugEsnVqVQxPEWyDWndQtSi2-6_uRlW_AeiDV6l1DSvFZkEQ9qPOatj3aH8QeoM8CxlNKIcjMGHCSyNBM5bkomODqIcIuxkrwQkrAit0MOnIIeYFDdiAeTRk_V6WDAR1AzfMUhaJKAcgL9ZcFj20o1epZUcNrUkQCA2TuBa6nYQDRC5LIaw6kM9rs6rUMNeJD3eOjlU9tKb_Ba0krvg">