<table border="1" cellspacing="0" cellpadding="8">
<tr>
<th>Issue</th>
<td>
<a href=https://github.com/llvm/llvm-project/issues/54860>54860</a>
</td>
</tr>
<tr>
<th>Summary</th>
<td>
Bad codegen: when optimizing for armeabi-v7a target, clang did not consider `-fno-aligned-allocation` option.
</td>
</tr>
<tr>
<th>Labels</th>
<td>
new issue
</td>
</tr>
<tr>
<th>Assignees</th>
<td>
</td>
</tr>
<tr>
<th>Reporter</th>
<td>
chirsz-ever
</td>
</tr>
</table>
<pre>
Environment: NDK23
The `clang --version` output of my NDK23:
```
Android (7714059, based on r416183c1) clang version 12.0.8 (https://android.googlesource.com/toolchain/llvm-project c935d99d7cf2016289302412d708641d52d2f7ee)
```
My cflags:
```
-frtti -fexceptions -std=c++17 -fno-aligned-allocation -flto
```
When I compile a Android native shared library for armeabi-v7a, there is a type like this:
```cpp
class Mat4 {
public:
//... only some member functions
Mat4 operator*(const Mat4& other) const {
// ...
}
private:
float data_[4][4];
};
```
And there is the code using `Mat4`, where crashes ramdomly occurs.:
```
// m_VertData.mat1, m_mat1, mat2 and m_mat3 are Mat4
m_VertData.mat1 = m_mat1 * mat2 * m_mat3;
```
After checking the generated assembly code, I found that:
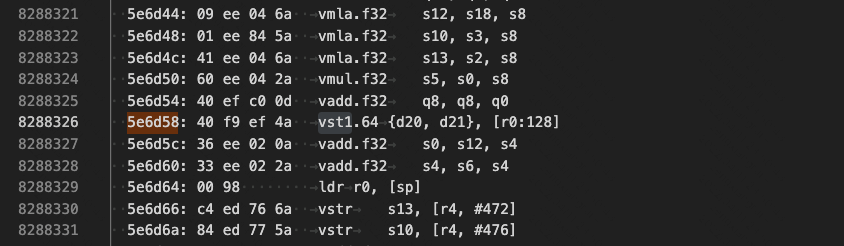
This `vst1.64 {d20, d21}, [r0:128]` requires `r0` with 16 bytes alignment, but acctually `r0` is not 16-byte-aligned.
My guess is that clang thinks `m_VertData.mat1` is 16-byte-aligned so generating the neon instructions, but due to the `-fno-aligned-allocation` option `m_VertData.mat1` is not 16-byte-aligned, and then the program crashed.
</pre>
<img width="1px" height="1px" alt="" src="http://email.email.llvm.org/o/eJyNVVGTozYM_jXkRQMDhkB4yEO2aWduOtenTvu4Y2wB7gKmtsk29-srG7K5y-xum2GCkS3pk_RJbrS8Hn-eLsroacTJRfkJfjv_yvIoPUfpaf3_vUeIylQMfOogji9orNITSUAvbl4c6BbG66aXn75XpUPbEz5PkzRaSYjYoaqyIt3XEfsJGm5Rgp7AFFmZHXKRRayG1d3mDDKWpMnBK_bOzda7Yb_Qw1eLSad1N6DVixGYCD3SltN6ED1XE62H4TLGs9F_oXAg6nwv61pWomVpVrJDnaesyJis0kNZZHLPJGsrRELxbhTr_9criHbgnf085Lg1zimIW_xH4OwoFguxdTLKzyJiT_RkFe1OOuaD6iaU9B604P4kyQenP8HwZ48TfAGKd1YDAodbgifSvyDYnhtK7aAaw80VWm2AmxF5o-JLxX3uXY8GQVnSddcZ6egLklB9GJWY51VC9bEWvnJXQFQ9rbJ5aQYl3lTB_9Y6JUlCFR6uYPWIMOLYoIF2mUTIyPeevE6wqmc03GkTMar1QdAxFzYiVoL2uANLgvgNAGy_1SmQ17s8qs4bSqMu3OEPMNtBcweSO_4c7Z-KaH--vfLNtFd_W79XDMr9PZ20IGwSYbGKaExHA3TSoKS_hlPCcNujBcNHqUdKjRZiMTb5nE9bZOPzH2jcmfAmI3eZtzo-v624Y0CdsYpyqjmumQsWHlSBmLjpUtpOq3JYBOX_iLl1VEfRo3jxYfqoO5x83Yh2xA-qMwXmE-FxfSEGLiFL3D1GyTJKuBp5hz7pj22-WDRx2LVJp1y_NF5CxXc0tLZ-z7OiKqqypKVv6jBg4rxq23TP2jgtcx4XiGl8KMt9XAlqcl6zQpRlMk_dvde3kUc1pFgv1mVJGRguWaidJKDEBFoRXpMSwowdPGQahwb_XpTBoGl8quCVsEJWQnN1JA4dHsasn3o0OLkQbqF-v941yO-kHenEXuc2FJKHydMtSM0XeEa0XUclde30Enw_kmM1-2CSOvFWq1vpJqSZo6ihzLL15YZTLjQUdDhDxj6YVuFCCBPuYwzvhOZ98LV1puCBxnRHPbH1xy30nTzmss5rvnPKDXh84jLwikLwl9ar1_beR_XNh_Mw6sBx02HI-5os6YckgfHzQ0ni8P-JK9ktZjj-yMyVjRsF_TXzcNvQp7KWykWLfXEo011_RAoDy7SsJU_rMi8K0VZNmzVt27CUy2I38AYHeySGRYxN-ArBBK2JaDt1ZCljKV1YROwyL5M8o88851m7P7AUWVSkOHI1JB5Hok23M8cAqVnotirSQVln75vUpj5kDO7IPl9cr81R9MrYbzHSDbwL7o8B_r_FHXUt">